How To Move Record From One Table To Another In Laravel
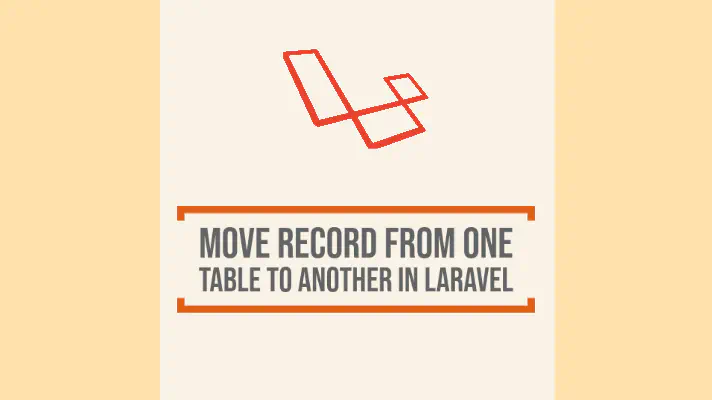
Laravel does not have any direct method to move an entire record from one table to another. It can be done indirectly. Let’s see one of the ways here in this article.
Laravel has a supporting feature for replicating or copying an entire row with the power of Model
in an optimized manner.
Laravel has an inbuilt method replicate()
of Model that can be used to prepare an entirely new, non-existing cloned instance of a model.
The save()
method will store this new clone model into the database.
Tested with Laravel version 6 and 7.
Kindly check for your version on the official DOC for Replicating Models. This link will take you to Laravel 7 Documentation.
Example
- In this example, assuming a use case where we want to move all those users whose last log is more than 3 years. Assuming these users as Inactive users.
- We will follow the below steps:
- Create a new instance with
replicate()
method based on the old or original record. - Store this new instance with
save()
method andsetTable()
method in the new table. - Delete the old record from the original table with
delete()
method of a model.
- Create a new instance with
Users::query()
->where('last_login','>', now()->subYears(3))
->each(function ($oldRecord) {
$newRecord = $oldRecord->replicate();
$newRecord->setTable('inactive_users');
$newRecord->save();
$oldRecord->delete();
});
This will move all records from users
table to inactive_users
who has not sign in into last 3 years
With method chaining
$oldRecord->replicate()
->setTable('inactive_users')
->save();
We also came across this query raised by the developer at Laracast forum and reply worth checking by willsmanley.
Resources
Happy 😄 coding