Identify whether the number is Even or Odd in C-language
If any number is exactly divisible by 2 then it’s an even number else it’s an odd number.
In this tutorial we’ll see how to check whether the provided number is even or odd number using Modulo(%
).
Flowchart
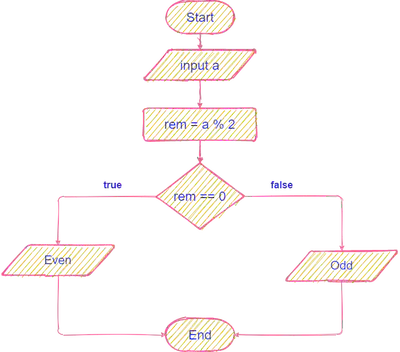
Note
- The remainder calculated with
%
(Modulo) on dividing by 2, will always be0
for all even numbers. - Whereas the remainder on dividing by 2, will always be
1
for all odd numbers.
Code
int main()
{
int a = 256;
int rem = a % 2;
printf("\nA: %d", a);
printf("\nRemainder: %d", rem);
if(rem == 0)
{
printf("\n%d is an even number", a);
}
else
{
printf("\n%d is an odd number", a);
}
/*
Divided by 2
12 % 2 -> 0
13 % 2 -> 1
14 % 2 -> 0
15 % 2 -> 1
16 % 2 -> 0
17 % 2 -> 1
18 % 2 -> 0
19 % 2 -> 1
20 % 2 -> 0
21 % 2 -> 1
22 % 2 -> 0
*/
printf("\n");
return 0;
}
Output
256 is an even number
Happy 😄 coding