How to find the length of a string using strlen() in C
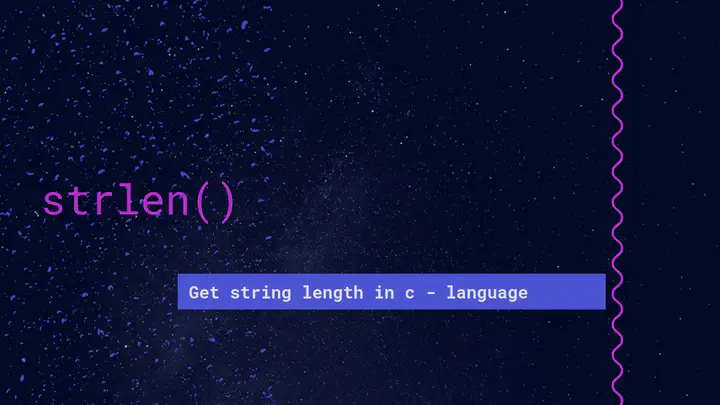
A tutorial for finding the length of a string using strlen in C - language
- The
strlen()
function counts the number of characters in a given string and returns the long unsigned integer value. - It is defined in C standard library, within
<string.h>
header file.
char proudToBeAnIndian[] = "East or West INDIA is best";
strlen(proudToBeAnIndian);
- It stops counting when the null character is encounter. Because in C, null character is considered as the end of the string.
Working example
#include <string.h>
#include <stdio.h>
int main()
{
char planet[] = "Earth", title[] = "MeshWorld";
printf("\n Using strlen for title without null character: %zu", strlen(title));
printf("\n Using sizeof for title with null character: %zu", sizeof(title));
printf("\n Using strlen for planet without null character: %zu", strlen(planet));
printf("\n Using sizeof for planet with null character: %zu", sizeof(planet));
return 0;
}
Output
Using strlen for title without null character: 9
Using sizeof for title with null character: 10
Using strlen for planet without null character: 5
Using sizeof for planet with null character: 6
Note that the strlen()
function doesn’t count for the null character \0
whereas sizeof()
function does.
Happy 😄 coding
With ❤️ from 🇮🇳