How To Drop Composite Indexes With Migration In Laravel
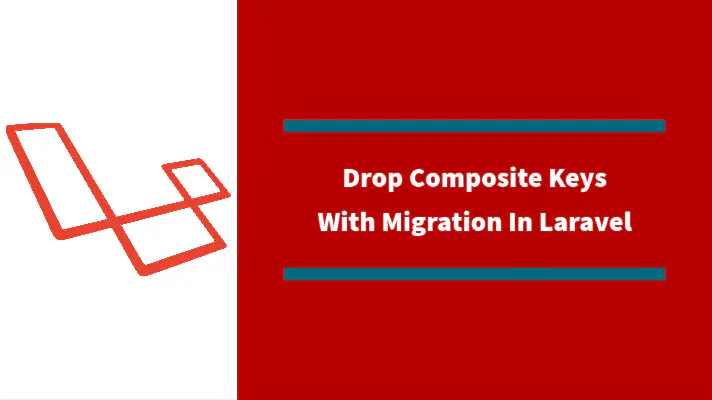
In previous article we saw [How to create composite keys with migration in Laravel]https://meshworld.in/create-composite-indexes-with-migration-in-laravel/.
In this tutorial we’ll see how to drop composite or compound index that is generated by Laravel using index
method with migration
.
Tested with Laravel version 5.8, 6 and 7.
Kindly check for your version on the official DOC for migrations. The DOC link will take you to Laravel 7.
Syntax
$table->dropIndex(['column1', 'column2']);
- For dropping composite key, we need to pass
array
type of parameter todropIndex
method. - We’ve specified our both columns for which we want to drop compound index.
- This method is useful when indexes is created by passing an array of columns into an
index
method. - The conventional key name will be based on the table name, columns and key type that will be used to drop previously generated indexes.
Example
- In this example we’re assuming that we already have
users
table withemail
andphone_number
fields. - The
up
method consists the code for creating the composite key. - And
down
method consists the code that will be used during migration rollback for dropping the composite key. - We’ve prepared
AlterUsersTable
migration with below code
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class AlterUsersTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up(): void
{
Schema::table('users', function (Blueprint $table) {
$table->index(['email', 'phone_number']);
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down(): void
{
Schema::table($this->tableName, function (Blueprint $table) {
$table->dropIndex(['email', 'phone_number']);
});
}
}
After executing php artisan migrate
, Laravel will update the migration and will take care of generating compound index.
Same way if executing php artisan migrate:rollback
, Laravel will downgrade the migration and will take care of dropping previously generated compound key.
Laravel will implicitly create compound/composite key with name users_email_phone_number_index
, based on table name and our specified fields. And same key will be used for dropping.
Note
- Laravel will automatically create and name our index key based table and our specified fields. We’re not explicitly specifying index name here.
Read our article about How to create composite key
with migration in Laravel
Happy coding