Programs Worth Practising for Absolute Beginners
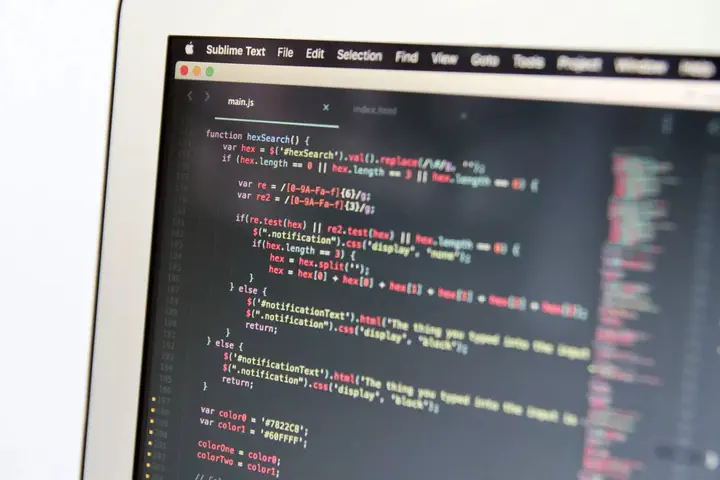
Following programs are worth practicing for absolute beginners. As someone starts the programming, these programs help to make hands on the syntax of the language as well as work towards improving their logic and problem-solving abilities.
Basic Input Output
- Write a program(WAP) to print “Hello World”.
- WAP to take user input such as name or any number and display it again on the screen.
- WAP to find the sum, difference, product, ratio, the remainder of two numbers with integers.
- WAP to find the sum, difference, product, ratio, the remainder of two numbers with decimal numbers.
- WAP to take user input and find the area of a rectangle.
- WAP to take user input and find the area of a triangle.
- WAP to take user input and find the area of a circle.
- WAP to take user input for Fahrenheit and convert it to Celsius (℉ to ℃).
- WAP to take user input for Celsius and convert it to Fahrenheit (℃ to ℉).
- WAP to take user input for mm(millimeter) and convert it to cm(centimeter).
- WAP to take user input for cm(centimeter) and convert it to mm(millimeter).
- WAP to take user input for angles in degrees and convert it to radians. Formulae is provided below. Either take value for PI as
22/7
or useMath.PI
available inMath
package in JAVA for example. - My electricity bills for the last three months have been
$23
,$32
and$64
. What is the average monthly electricity bill over the three month period? Write an expression to calculate themean
and display respective result.
radians = degrees * PI/180
- WAP to print ASCII value of a given input character.
- WAP to take user input and display the type of data entered by a user.
- WAP to find the size of different data types on your machine
- WAP to calculate sum and average of three numbers.
- WAP to swap two numbers using a temporary or third variable.
- WAP to swap two numbers without using the temporary or third variable.
- WAP that illustrates type conversion i.e converting integer to decimal(int to float)
Using ‘if-else’
, ‘switch’
and different operators
- WAP to take user input for two number and find greater of them.
- WAP to take user input for a number and find whether it is positive or negative.
- WAP to take user input for a number and find whether it is zero or non-zero.
- WAP to take user input for two variables and find whether both are same or different.
- WAP to take user input for a number and find whether it is odd or even.
- WAP to take user input for a year and find whether it is a leap year or not.
- WAP to take user input for a number and find whether it is prime or not.
- WAP to take user input for a number and find whether it is perfect or not.
- WAP to take user input for a number and find whether it is divisible by 5 or not.
- WAP to take user input and find whether it is an alphabet or not.
- WAP to take user input and find whether it is an alphanumeric or not.
- WAP to take user input for a character and find whether it is a vowel or a consonant.
- WAP to take user input for three variables(numbers) and find the greatest, of all three of them, using NESTED IF.
- WAP to take user input for three variables(numbers) and find the greatest, of all three of them using Logical Operators within IF.
- WAP to take user input for cost price and selling price and identify whether the seller is in profit or loss.
Do all programs from within Using ‘if-else’, ‘switch’ and different operators: section using ternary operator(? :)
- WAP to calculate roots of a quadratic equation.
- WAP to find LCM and HCF of two given numbers.
- WAP to make a calculator using a switch.
- WAP to find if a character is in uppercase, lowercase, digit or special character using a switch.
Using Loops
WAP to print numbers from 1 to n using different loops.
WAP to print numbers from n to 1 using different loops.
WAP to print odd series between two numbers of user choice using different loops.
WAP to print even series between two numbers of user choice using different loops.
WAP to find the factorial of a given number using different loops.
WAP to find the sum of series using different loops.
WAP to print the following pattern up to N lines:- ( e.g : 4 )
* * * * * * * * * *
WAP to print the following pattern up to N lines:- ( e.g : 4 )
* * * * * * * * * *
WAP to print the following pattern up to N lines:- ( e.g : 4 )
1 2 2 3 3 3 4 4 4 4
WAP to print the following pattern up to N lines:- ( e.g : 4 )
1 1 2 1 2 3 1 2 3 4
WAP to print a triangle of stars(
‘*’
) up to N lines.//Triangle pattern * * * * * * * * * * * * * * * *
WAP to print an inverted triangle of stars(
‘*’
) up to N lines.//Inverted triangle pattern * * * * * * * * * * * * * * * *
WAP to print the following pattern up to N lines ( e.g : 10 )
0 0 1 0 2 4 0 3 6 9 0 4 8 12 16 0 5 10 15 20 25 0 6 12 18 24 30 36 0 7 14 21 28 35 42 49 0 8 16 24 32 40 48 56 64 0 9 18 27 36 45 54 63 72 81
WAP to count the total digits in a number.
WAP to print the sum of all digits in a number.
WAP to check if given string is Palindrome or not.
WAP to check if a number is Armstrong or not.
Using Functions
- WAP using a recursive function to find the factorial of a number.
- WAP using a recursive function to find out the sum of natural numbers up to N.
Using Arrays
- WAP to print an array elements with each element double its value.
- WAP to calculate the sum of array elements both row-wise and column-wise.
- WAP to find largest and second largest in an array.
- WAP to sort an array using Insertion Sort.
- WAP to sort an array using Insertion Sort recursively.
- WAP to sort an array using Selection Sort.
- WAP to sort an array using Bubble Sort.
- WAP to sort an array using Bubble Sort recursively.
- WAP to sort an array using Merge Sort.
- WAP to sort an array using Merge Sort recursively.
- WAP to sort an array using Quick Sort.
- WAP to sort an array using Quick Sort recursively.
- WAP to search an element in an array using Binary Search Technique(BST).
- WAP to merge two sorted arrays.
- WAP to print a two-dimensional(2D) array both row-wise and column-wise.
- WAP to multiply two-dimensional arrays.
These programs will give you the head-start.
Hope you find this helpful. Happy coding!
Will keep updating these list.